In this How to pull the hashtags from the FeedBurner post, we have extracted the hashtags that we are going to post on the Facebook page, and in this post, you have learned How to get the Access Token for Facebook Graph API? we have generated the access token to publish the post on the Facebook page.
In this article, you will learn how to retrieve, publish, and delete the posts from the Facebook page.
In order to perform various actions on Facebook, there is a popular unofficial Python 3rd party library called Facebook-SDK which is a prerequisite for connecting to Facebook. To install the Facebook-SDK python library, use below commands in the command prompt as shown in 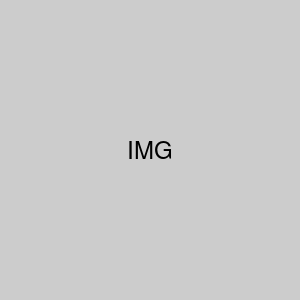
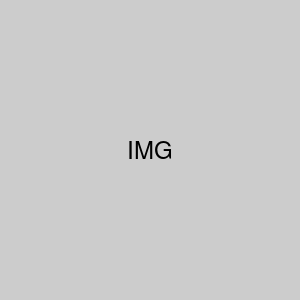
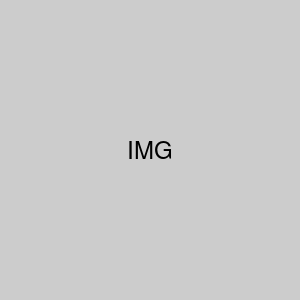
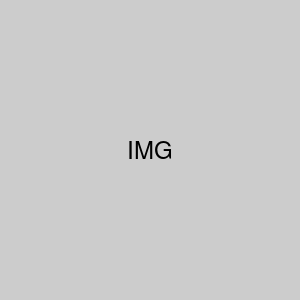
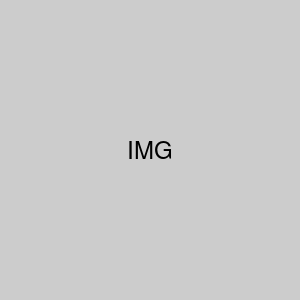
pip install facebook-sdk
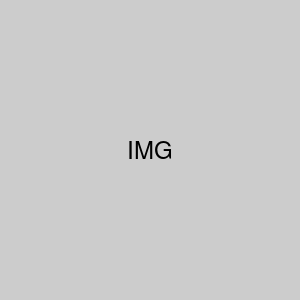
Since the Facebook-SDK library is already installed on my computer, therefore, I'm seeing the requirement already satisfied, In your case, you should see the installation complete and success message.
Copy the below code and save it with .py extension and replace the access token with your token.
How to connect to Facebook via Python script?
import feedparserThe output looks like this:
import re
import facebook
count=0
graph = facebook.GraphAPI(access_token="EAAqj5wU098QBAK0qbdFe8lDhf1eBBRKI2zxqiqzCkFEMVZCh5bECvPZCUNitH6jLHuodbGyHZBFp1ZBDCT2KRVTPDRg461xH3tek8E61M8XhPq4npuV8nVVNkrqIACW0aMrNNrjOBoIdP777Y4QjpWIVDIYZCZBVdCgp3m4FyimmeWq6DP2qVf3E7R7JV7bOaK06o4NmrT1bJw1F8GgVNARGOIDANt6WQEJUNNtmCyMQZDZD")
posts = graph.request('/me')
postslist = posts['name']
print(postslist)
NewsFeed = feedparser.parse("http://feeds.feedburner.com/Especiallysports")
for item in NewsFeed.entries:
print('Post Title :',item.title)
print('Post Link :',item.feedburner_origlink)
result = re.findall(r"#(\w+)", item.summary)
aces = ["#" + suit for suit in result]
hashtags = ' '.join(aces)
print('Post hashtags :',hashtags)
print('')
count=count+1
if count>2:
break
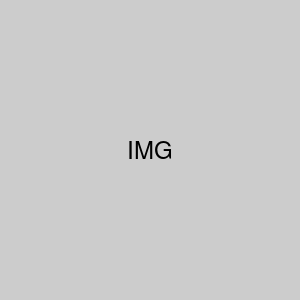
If you replace /me with your Facebook page ID, then it will display page name:
posts = graph.request('/Facebook-Page-ID')
How to retreive the Facebook page posts via Python script?
import feedparser import re import facebook count=0 #Faceook connection graph = facebook.GraphAPI(access_token="EAAqj5wU098QBAK0qbdFe8lDhf1eBBRKI2zxqiqzCkFEMVZCh5bECvPZCUNitH6jLHuodbGyHZBFp1ZBDCT2KRVTPDRg461xH3tek8E61M8XhPq4npuV8nVVNkrqIACW0aMrNNrjOBoIdP777Y4QjpWIVDIYZCZBVdCgp3m4FyimmeWq6DP2qVf3E7R7JV7bOaK06o4NmrT1bJw1F8GgVNARGOIDANt6WQEJUNNtmCyMQZDZD") posts = graph.request('/me') postslist = posts['name'] print(postslist) #retreiving hashtags from the feedburner and display recent 3 posts tile, link and hastags NewsFeed = feedparser.parse("http://feeds.feedburner.com/Especiallysports") for item in NewsFeed.entries: print('Post Title :',item.title) print('Post Link :',item.feedburner_origlink) result = re.findall(r"#(\w+)", item.summary) aces = ["#" + suit for suit in result] hashtags = ' '.join(aces) print('Post hashtags :',hashtags) print('') count=count+1 if count>2: break #getting all the posts from Facebook page feed = graph.get_connections("101913231469038", "feed") post = feed["data"] for item in post: print("item id is",item['id'], "item message", item['message'])
Output:
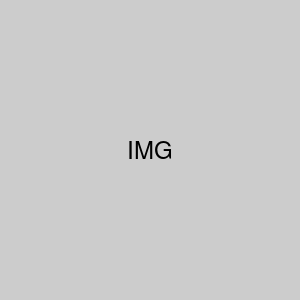
By default, the above script will throw 10 posts, to show only first 3 articles, you can use the below script
import feedparser
import re
import facebook
count=0
count1=0
#Faceook connection
graph = facebook.GraphAPI(access_token="EAAqj5wU098QBAK0qbdFe8lDhf1eBBRKI2zxqiqzCkFEMVZCh5bECvPZCUNitH6jLHuodbGyHZBFp1ZBDCT2KRVTPDRg461xH3tek8E61M8XhPq4npuV8nVVNkrqIACW0aMrNNrjOBoIdP777Y4QjpWIVDIYZCZBVdCgp3m4FyimmeWq6DP2qVf3E7R7JV7bOaK06o4NmrT1bJw1F8GgVNARGOIDANt6WQEJUNNtmCyMQZDZD")
posts = graph.request('/me')
postslist = posts['name']
print(postslist)
#retreiving hashtags from the feedburner and display recent 3 posts tile, link and hastags
NewsFeed = feedparser.parse("http://feeds.feedburner.com/Especiallysports")
for item in NewsFeed.entries:
print('Post Title :',item.title)
print('Post Link :',item.feedburner_origlink)
result = re.findall(r"#(\w+)", item.summary)
aces = ["#" + suit for suit in result]
hashtags = ' '.join(aces)
print('Post hashtags :',hashtags)
print('')
count=count+1
if count>2:
break
#getting all the posts from Facebook page
feed = graph.get_connections("101913231469038", "feed")
post = feed["data"]
for item in post:
print("item id is",item['id'], "item message", item['message'])
count1=count1+1
if count1>2:
break
Output: will show only 3 posts
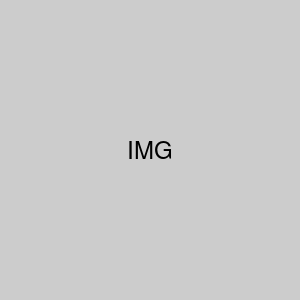
How to publish the posts on the Facebook page via Python Script?
import feedparser
import re
import facebook
count=0
count1=0
#Faceook connection
graph = facebook.GraphAPI(access_token="EAAqj5wU098QBAPXWzeZC9qFpA3meIwRcxYjHS3NMUddsMKL9F4ULjmI90eqblfuPz0ZB2ZCupob1hWBvy0ZAAoaP7ZAG2q7rDxxrXNVxZCMjrsVkZAKvvGGGZAyHRjKplh5OL5JBwkbpPHAim1TN9W1x8hIOgULjWtOiCO5dSZCS6ZAZA8vxSOLzGNQSqbnD1lLBOIDROUwZCwcZAngZDZD")
posts = graph.request('/101913231469038')
postslist = posts['name']
print(postslist)
#retreiving hashtags from the feedburner and display recent 3 posts tile, link and hastags
NewsFeed = feedparser.parse("http://feeds.feedburner.com/Especiallysports")
for item in NewsFeed.entries:
print('Post Title :',item.title)
print('Post Link :',item.feedburner_origlink)
result = re.findall(r"#(\w+)", item.summary)
aces = ["#" + suit for suit in result]
hashtags = ' '.join(aces)
print('Post hashtags :',hashtags)
#publishing posts on Facebook page
newpost=graph.put_object("101913231469038", "feed", message=hashtags,link=item.feedburner_origlink)
print(newpost['id'])
print('')
count=count+1
if count>2:
break
#getting all the posts from Facebook page
feed = graph.get_connections("101913231469038", "feed")
post = feed["data"]
for item in post:
print("item id is",item['id'], "item message", item['message'])
count1=count1+1
if count1>2:
break
Output:
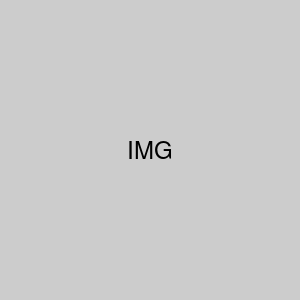
Below are new posts are that are published on the Facebook page.
How to delete the posts on the Facebook page via Python Script?
import feedparser
import re
import facebook
count=0
count1=0
#Faceook connection
graph = facebook.GraphAPI(access_token="EAAqj5wU098QBAPXWzeZC9qFpA3meIwRcxYjHS3NMUddsMKL9F4ULjmI90eqblfuPz0ZB2ZCupob1hWBvy0ZAAoaP7ZAG2q7rDxxrXNVxZCMjrsVkZAKvvGGGZAyHRjKplh5OL5JBwkbpPHAim1TN9W1x8hIOgULjWtOiCO5dSZCS6ZAZA8vxSOLzGNQSqbnD1lLBOIDROUwZCwcZAngZDZD")
posts = graph.request('/101913231469038')
postslist = posts['name']
print(postslist)
#retreiving hashtags from the feedburner and display recent 3 posts tile, link and hastags
NewsFeed = feedparser.parse("http://feeds.feedburner.com/Especiallysports")
for item in NewsFeed.entries:
print('Post Title :',item.title)
print('Post Link :',item.feedburner_origlink)
result = re.findall(r"#(\w+)", item.summary)
aces = ["#" + suit for suit in result]
hashtags = ' '.join(aces)
print('Post hashtags :',hashtags)
#publishing posts on Facebook page
newpost=graph.put_object("101913231469038", "feed", message=hashtags,link=item.feedburner_origlink)
print(newpost['id'])
print('')
count=count+1
if count>2:
break
#getting all the posts from Facebook page
feed = graph.get_connections("101913231469038", "feed")
post = feed["data"]
for item in post:
print("item id is",item['id'], "item message", item['message'])
count1=count1+1
if count1>3:
break
#delete the required post with user input
userinput= input("do you want to delete the post")
if userinput=='yes':
if graph.delete_object(item['id']):
print("successfully deleted")
Hope this article is helpful
Happy Learning :)
EmoticonEmoticon